How to export image annotations and sample export formats.
Export as JSON
# Set the export params to include/exclude certain fields.
export_params= {
"attachments": True,
"metadata_fields": True,
"data_row_details": True,
"project_details": True,
"label_details": True,
"performance_details": True,
"interpolated_frames": True
}
# Note: Filters follow AND logic, so typically using one filter is sufficient.
filters= {
"last_activity_at": ["2000-01-01 00:00:00", "2050-01-01 00:00:00"],
"workflow_status": "<wkf-status>"
}
export_task = project.export(params=export_params, filters=filters)
export_task.wait_till_done()
# Stream the export using a callback function
def json_stream_handler(output: labelbox.BufferedJsonConverterOutput):
print(output.json)
export_task.get_buffered_stream(stream_type=labelbox.StreamType.RESULT).start(stream_handler=json_stream_handler)
# Collect all exported data into a list
export_json = [data_row.json for data_row in export_task.get_buffered_stream()]
print("file size: ", export_task.get_total_file_size(stream_type=lb.StreamType.RESULT))
print("line count: ", export_task.get_total_lines(stream_type=lb.StreamType.RESULT))
Annotation export formats
Bounding box
{
"feature_id": "clrf5csvi6ofm07lsf9pygwvi",
"feature_schema_id": "clrf5ck4a0b9b071paa9ncu15",
"name": "bounding_box",
"annotation_kind": "ImageBoundingBox",
"classifications": [],
"bounding_box": {
"top": 1264.0,
"left": 2096.0,
"height": 425.0,
"width": 144.0
}
}
Polygon
{
"feature_id": "clr0x0ol83tvt07ea4has2a4l",
"feature_schema_id": "clpk2me9t02v707xn08cv4ve5",
"name": "polygon",
"annotation_kind": "ImagePolygon",
"classifications": [],
"polygon": [
{
"x": 1489.581,
"y": 183.934
},
{
"x": 1489.581,
"y": 183.934
}
]
}
Polyline
{
"feature_id": "clr0x0ol83tvk07eagehegjde",
"feature_schema_id": "clpk2me9u02vh07xnfcmtbqvj",
"name": "polyline",
"annotation_kind": "ImagePolyline",
"classifications": [],
"line": [
{
"x": 2534.353,
"y": 249.471
},
{
"x": 90.929,
"y": 326.412
}
]
}
Point
{
"feature_id": "clr0x0ol83tvr07ea2fyl69g9",
"feature_schema_id": "clpk2me9u02vf07xnbpk45wxa",
"name": "point",
"annotation_kind": "ImagePoint",
"classifications": [],
"point": {
"x": 1166.606,
"y": 1441.768
}
}
Mask
Regardless of which tool is used to create a segmentation mask, the annotation will appear in the following form in the export. Whether you use AutoSegment, the brush tool, the pen tool, or the fill tool, all masks take the same format in exports.
Composite masks
Open this Colab for an interactive tutorial on exporting composite masks.
A composite mask is a cloud-hosted PNG file that contains all raster segmentation mask instances on a labeled data row (one composite mask per label).
This screenshot shows an example composite mask in PNG format. Here, the image has eight segmentation mask instances, each using the schema named "Human".
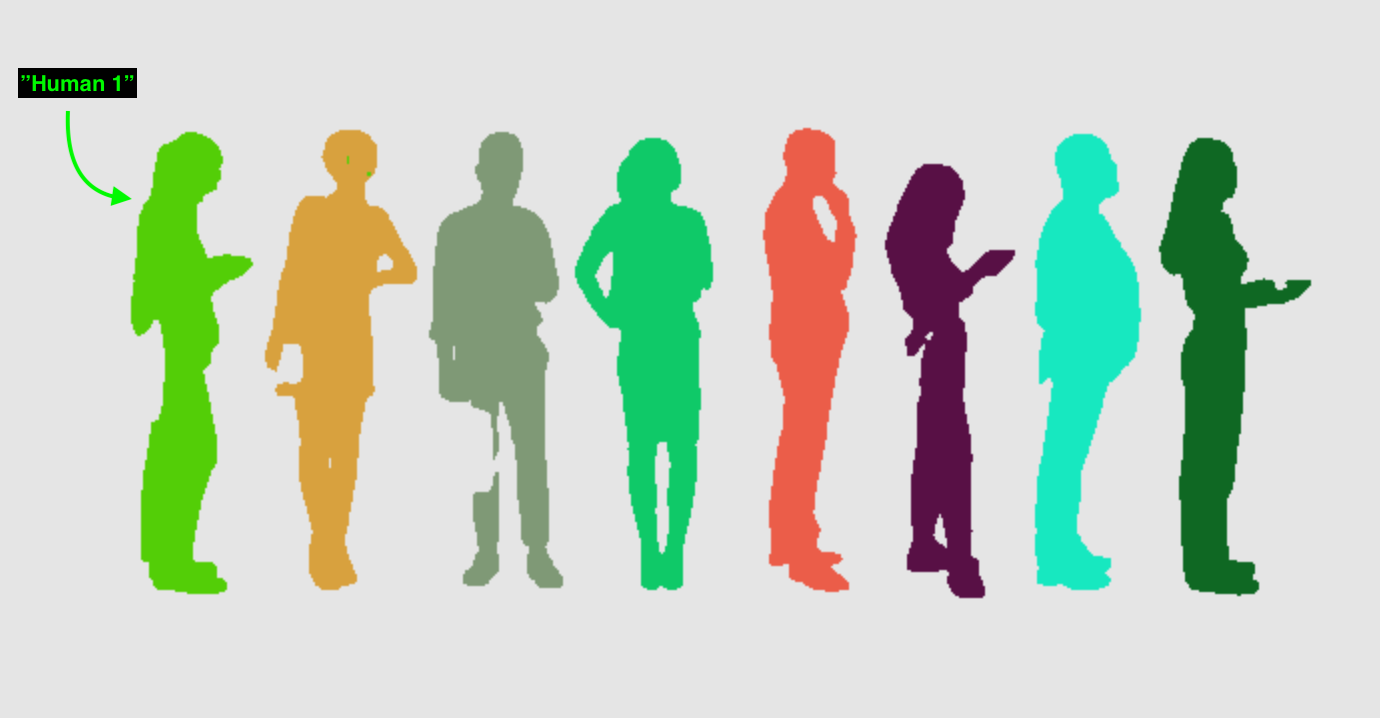
A labeled data row containing 8 instances of a segmentation mask named "Human".
When you export data rows containing segmentation masks, you can use composite_mask.url
to access all segmentation masks on that data row. To access a single instance of a segmentation mask, use the color_rgb
values. The color_rgb
value represents the color of a single annotation or instance mask in a label.
Sample composite masks export
{
"feature_id": "clsivokxg00043b70xuf7x5tz",
"feature_schema_id": "clsgbcaqw000j071t2ga23jnc",
"name": "car",
"value": "car",
"annotation_kind": "ImageSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/clsivnieg050k074i2jqaa0c3/annotations/clsivokxg00043b70xuf7x5tz/index/1/mask"
},
"composite_mask": {
// This URL contains all mask instances on the data row
"url": "https://api.labelbox.com/api/v1/tasks/clsiw88n205mw072ke4n78y5o/masks/clsivok5f00023b70r1a4fgzy",
// This RGB reflects a single mask instance
"color_rgb": [
31,
185,
101
]
}
}
Access mask data
To access the mask data, you must pass your Labelbox API key stored in client.headers
in an API request.
When you grab a URL from the mask annotation in the export, the project_id
and feature_id
will already be in place, as shown above. Here, we provide the framework for structuring a URL with any project ID and feature ID.
import urllib.request
from PIL import Image
# Provide a project ID and feature ID if using instance masks. Alternatively, replace the entire mask_url with a URL grabbed from your export.
project_id = ""
feature_id = ""
mask_url = f"https://api.labelbox.com/api/v1/projects/{project_id}/annotations/{feature_id}/index/1/mask"
# Instead of providing the individual variables, we recommend utilizing the URL from export for composite mask
composite_mask_url = f"https://api.labelbox.com/api/v1/tasks/{task_id}/masks/{composite_mask_id}/index/1"
# Make the API request
req = urllib.request.Request(mask_url, headers=client.headers)
# Optionally, print the image of the mask
image = Image.open(urllib.request.urlopen(req))
image
Relationship
{
"feature_id": "clur69pfa00023b6rkftlkucz",
"feature_schema_id": "cltd3vkc201ab07ts1f9dhpkt",
"name": "relationship",
"value": "relationship",
"annotation_kind": "ImageUnidirectionalRelationship",
"classifications": [],
"unidirectional_relationship": {
"source": "clur5tlyq1n2d07dcg9xldg90",
"target": "clur5tlyr1n2l07dc1jsf15qo"
}
}
Classification - radio
{
"feature_id": "clur5tlyr1n2k07dcezk08npu",
"feature_schema_id": "cltd3vkc201ad07ts3t78873p",
"name": "radio_question",
"value": "radio_question",
"radio_answer": {
"feature_id": "clur5tlyr1n2y07dcajpyg0d4",
"feature_schema_id": "cltd3vkc201ag07tsfoc2bdpi",
"name": "second_radio_answer",
"value": "second_radio_answer",
"classifications": []
}
}
Classification - checklist
{
"feature_id": "clur5tlyr1n2p07dchs791672",
"feature_schema_id": "cltd3vkc201aj07tsait4cbzh",
"name": "checklist_question",
"value": "checklist_question",
"checklist_answers": [
{
"feature_id": "clur5tlyr1n2z07dc3abv19e8",
"feature_schema_id": "cltd3vkc201ak07tsfqxc740z",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "clur5tlyr1n3007dc4vbr0628",
"feature_schema_id": "cltd3vkc201am07ts7s9e7x7k",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
}
Classification - free-form text
{
"feature_id": "clur5tlyr1n2g07dc7wdv7p5z",
"feature_schema_id": "cltd3vkc201ap07ts58gma0bb",
"name": "free_text",
"value": "free_text",
"text_answer": {
"content": "sample text"
}
}
Sample project export
{
"data_row": {
"id": "clf9spzxq029m071nehy60a6v",
"global_key": "2560px-Kitano_Street_Kobe01s5s4110.jpeg",
"row_data": "https://storage.googleapis.com/labelbox-datasets/image_sample_data/2560px-Kitano_Street_Kobe01s5s4110.jpeg",
"details": {
"dataset_id": "clf9spwgw04w207ymfwy826qv",
"dataset_name": "demo_dataset_img",
"created_at": "2023-03-15T14:46:58.000+00:00",
"updated_at": "2023-03-15T14:46:58.000+00:00",
"last_activity_at": "2024-04-08T16:34:01.000+00:00",
"created_by": "labelbox.public.user@labelbox.com"
}
},
"media_attributes": {
"height": 1707,
"width": 2560,
"mime_type": "image/jpeg"
},
"attachments": [],
"metadata_fields": [],
"embeddings": [
{
"id": "cn1cywy1r077807zblvo0m884",
"name": "my_custom_embedding_2048_dimensions",
"dimensions": 2048,
"is_custom": true,
"values": [
{
"value": [
1.1265901290930636,
1.0413733599834287,
1.3041214120015427,
1.893491997791636 ...
]
}
]
},
{
"id": "c200000000000000000000000",
"name": "Image Embedding V2 (CLIP ViT-B/32)",
"dimensions": 512,
"is_custom": false,
"values": [
{
"value": [
-0.4192526042461395,
-0.048827216029167175 ...
]
}
]
}
],
"projects": {
"cltd3vkju021907u63w2odj69": {
"name": "Image Annotation Import Demo",
"labels": [
{
"label_kind": "Default",
"version": "1.0.0",
"id": "clur5tlda1n2a07dc0i0zdd4k",
"label_details": {
"created_at": "2024-04-08T16:21:26.000+00:00",
"updated_at": "2024-04-08T16:34:02.000+00:00",
"created_by": "user@labelbox.com",
"content_last_updated_at": "2024-04-08T16:34:00.513+00:00",
"reviews": []
},
"performance_details": {
"seconds_to_create": 18,
"seconds_to_review": 6,
"skipped": false
},
"annotations": {
"objects": [
{
"feature_id": "clur5tlyq1n2d07dcg9xldg90",
"feature_schema_id": "cltd3vkc1019r07ts2lru4hi4",
"name": "bounding_box",
"value": "bounding_box",
"annotation_kind": "ImageBoundingBox",
"classifications": [],
"bounding_box": {
"top": 1264.0,
"left": 2096.0,
"height": 425.0,
"width": 144.0
}
},
{
"feature_id": "clur5tlyr1n2f07dcf78sa4y5",
"feature_schema_id": "cltd3vkc201a707ts9ullbhfo",
"name": "point",
"value": "point",
"annotation_kind": "ImagePoint",
"classifications": [],
"point": {
"x": 1166.606,
"y": 1441.768
}
},
{
"feature_id": "clur5tlyr1n2h07dc1pxnhoj3",
"feature_schema_id": "cltd3vkc1019t07ts2bk6hjjj",
"name": "bbox_with_radio_subclass",
"value": "bbox_with_radio_subclass",
"annotation_kind": "ImageBoundingBox",
"classifications": [
{
"feature_id": "clur5tlyr1n2v07dcbnnfh1ah",
"feature_schema_id": "cltd3vkc1019u07tsaari5i2k",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "clur5tlyr1n3607dc98qpagxb",
"feature_schema_id": "cltd3vkc1019v07tsbow08f9u",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
],
"bounding_box": {
"top": 933.0,
"left": 541.0,
"height": 191.0,
"width": 330.0
}
},
{
"feature_id": "clur5tlyr1n2j07dc50cs4e11",
"feature_schema_id": "cltd3vkc201a907ts69dr5m86",
"name": "polyline",
"value": "polyline",
"annotation_kind": "ImagePolyline",
"classifications": [],
"line": [
{
"x": 2534.353,
"y": 249.471
},
{
"x": 2429.492,
"y": 182.092
},
{
"x": 2294.322,
"y": 221.962
},
{
"x": 2224.491,
"y": 180.463
},
{
"x": 2136.123,
"y": 204.716
},
{
"x": 1712.247,
"y": 173.949
},
{
"x": 1703.838,
"y": 84.438
},
{
"x": 1579.772,
"y": 82.61
},
{
"x": 1583.442,
"y": 167.552
},
{
"x": 1478.869,
"y": 164.903
},
{
"x": 1418.941,
"y": 318.149
},
{
"x": 1243.128,
"y": 400.815
},
{
"x": 1022.067,
"y": 319.007
},
{
"x": 892.367,
"y": 379.216
},
{
"x": 670.273,
"y": 364.408
},
{
"x": 613.114,
"y": 288.16
},
{
"x": 377.559,
"y": 238.251
},
{
"x": 368.087,
"y": 185.064
},
{
"x": 246.557,
"y": 167.286
},
{
"x": 236.648,
"y": 285.61
},
{
"x": 90.929,
"y": 326.412
}
]
},
{
"feature_id": "clur5tlyr1n2l07dc1jsf15qo",
"feature_schema_id": "cltd3vkc1019r07ts2lru4hi4",
"name": "bounding_box",
"value": "bounding_box",
"annotation_kind": "ImageBoundingBox",
"classifications": [],
"bounding_box": {
"top": 1346.0,
"left": 2272.0,
"height": 358.0,
"width": 144.0
}
},
{
"feature_id": "clur5tlyr1n2m07dc4x6xhzep",
"feature_schema_id": "cltd3vkc1019z07ts7g1n3o5m",
"name": "polygon",
"value": "polygon",
"annotation_kind": "ImagePolygon",
"classifications": [],
"polygon": [
{
"x": 1489.581,
"y": 183.934
},
{
"x": 2278.306,
"y": 256.885
},
{
"x": 2428.197,
"y": 200.437
},
{
"x": 2560.0,
"y": 335.419
},
{
"x": 2557.386,
"y": 503.165
},
{
"x": 2320.596,
"y": 503.103
},
{
"x": 2156.083,
"y": 628.943
},
{
"x": 2161.111,
"y": 785.519
},
{
"x": 2002.115,
"y": 894.647
},
{
"x": 1838.456,
"y": 877.874
},
{
"x": 1436.53,
"y": 874.636
},
{
"x": 1411.403,
"y": 758.579
},
{
"x": 1353.853,
"y": 751.74
},
{
"x": 1345.264,
"y": 453.461
},
{
"x": 1426.011,
"y": 421.129
},
{
"x": 1489.581,
"y": 183.934
}
]
},
{
"feature_id": "clur5tlyr1n2n07dc4qot5gbx",
"feature_schema_id": "cltd3vkc1019r07ts2lru4hi4",
"name": "bounding_box",
"value": "bounding_box",
"annotation_kind": "ImageBoundingBox",
"classifications": [],
"bounding_box": {
"top": 977.0,
"left": 1690.0,
"height": 330.0,
"width": 225.0
}
},
{
"feature_id": "clur5tlyr1n2q07dc5joc4k7u",
"feature_schema_id": "cltd3vkc101a107ts95sk63u0",
"name": "mask",
"value": "mask",
"annotation_kind": "ImageSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd3vkju021907u63w2odj69/annotations/clur5tlyr1n2q07dc5joc4k7u/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6dp8r04ul07wrgp9q4pla/masks/clur5tlyr1n2o07dc99lw3r8p/index/1",
"color_rgb": [
10,
10,
125
]
}
},
{
"feature_id": "clur5tlyr1n2r07dc6ym4d4mc",
"feature_schema_id": "cltd3vkc201a307tsforlev07",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "ImageSegmentationMask",
"classifications": [
{
"feature_id": "clur5tlyr1n2x07dc84a12l8i",
"feature_schema_id": "cltd3vkc201a407tsfo3agh7x",
"name": "sub_free_text",
"value": "sub_free_text",
"text_answer": {
"content": "free text answer sample"
}
}
],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd3vkju021907u63w2odj69/annotations/clur5tlyr1n2r07dc6ym4d4mc/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6dp8r04ul07wrgp9q4pla/masks/clur5tlyr1n2o07dc99lw3r8p/index/1",
"color_rgb": [
105,
136,
97
]
}
},
{
"feature_id": "clur5tlyr1n2s07dc739a6kqs",
"feature_schema_id": "cltd3vkc201a307tsforlev07",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "ImageSegmentationMask",
"classifications": [
{
"feature_id": "clur5tlyr1n2w07dccdv0g7gg",
"feature_schema_id": "cltd3vkc201a407tsfo3agh7x",
"name": "sub_free_text",
"value": "sub_free_text",
"text_answer": {
"content": "free text answer sample"
}
}
],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd3vkju021907u63w2odj69/annotations/clur5tlyr1n2s07dc739a6kqs/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6dp8r04ul07wrgp9q4pla/masks/clur5tlyr1n2o07dc99lw3r8p/index/1",
"color_rgb": [
7,
85,
11
]
}
},
{
"feature_id": "clur5tlyr1n2t07dc0oxlgoc8",
"feature_schema_id": "cltd3vkc201a307tsforlev07",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "ImageSegmentationMask",
"classifications": [
{
"feature_id": "clur5tlyr1n3107dccy5pbudr",
"feature_schema_id": "cltd3vkc201a407tsfo3agh7x",
"name": "sub_free_text",
"value": "sub_free_text",
"text_answer": {
"content": "free text answer sample"
}
}
],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd3vkju021907u63w2odj69/annotations/clur5tlyr1n2t07dc0oxlgoc8/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6dp8r04ul07wrgp9q4pla/masks/clur5tlyr1n2o07dc99lw3r8p/index/1",
"color_rgb": [
35,
34,
212
]
}
},
{
"feature_id": "clur5tlyr1n2u07dc5wjmdm3v",
"feature_schema_id": "cltd3vkc101a107ts95sk63u0",
"name": "mask",
"value": "mask",
"annotation_kind": "ImageSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd3vkju021907u63w2odj69/annotations/clur5tlyr1n2u07dc5wjmdm3v/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6dp8r04ul07wrgp9q4pla/masks/clur5tlyr1n2o07dc99lw3r8p/index/1",
"color_rgb": [
232,
194,
86
]
}
}
],
"classifications": [
{
"feature_id": "clur5tlyr1n2e07dc2bez8p1u",
"feature_schema_id": "cltd3vkc201ar07ts3gq6a6sn",
"name": "nested_radio_question",
"value": "nested_radio_question",
"radio_answer": {
"feature_id": "clur5tlyr1n3307dcexth5ff7",
"feature_schema_id": "cltd3vkc201as07tsh2prgosu",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": [
{
"feature_id": "clur5tlyr1n3407dc306b5xib",
"feature_schema_id": "cltd3vkc201at07tsdjx9dlbw",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "clur5tlyr1n3507dcef304jmb",
"feature_schema_id": "cltd3vkc201au07ts7nk47qny",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
]
}
},
{
"feature_id": "clur5tlyr1n2g07dc7wdv7p5z",
"feature_schema_id": "cltd3vkc201ap07ts58gma0bb",
"name": "free_text",
"value": "free_text",
"text_answer": {
"content": "sample text"
}
},
{
"feature_id": "clur5tlyr1n2i07dcd8lx87be",
"feature_schema_id": "cltd3vkc201az07ts7st3fn79",
"name": "nested_checklist_question",
"value": "nested_checklist_question",
"checklist_answers": [
{
"feature_id": "clur5tlyr1n3207dc4u4nfg08",
"feature_schema_id": "cltd3vkc201b007tsdhs07baf",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": [
{
"feature_id": "clur5tlyr1n3707dc2cy805ac",
"feature_schema_id": "cltd3vkc201b107ts12b1al1j",
"name": "sub_checklist_question",
"value": "sub_checklist_question",
"checklist_answers": [
{
"feature_id": "clur5tlyr1n3807dcgpgih5qj",
"feature_schema_id": "cltd3vkc201b207ts434b9iyx",
"name": "first_sub_checklist_answer",
"value": "first_sub_checklist_answer",
"classifications": []
}
]
}
]
}
]
},
{
"feature_id": "clur5tlyr1n2k07dcezk08npu",
"feature_schema_id": "cltd3vkc201ad07ts3t78873p",
"name": "radio_question",
"value": "radio_question",
"radio_answer": {
"feature_id": "clur5tlyr1n2y07dcajpyg0d4",
"feature_schema_id": "cltd3vkc201ag07tsfoc2bdpi",
"name": "second_radio_answer",
"value": "second_radio_answer",
"classifications": []
}
},
{
"feature_id": "clur5tlyr1n2p07dchs791672",
"feature_schema_id": "cltd3vkc201aj07tsait4cbzh",
"name": "checklist_question",
"value": "checklist_question",
"checklist_answers": [
{
"feature_id": "clur5tlyr1n2z07dc3abv19e8",
"feature_schema_id": "cltd3vkc201ak07tsfqxc740z",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "clur5tlyr1n3007dc4vbr0628",
"feature_schema_id": "cltd3vkc201am07ts7s9e7x7k",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
}
],
"relationships": [
{
"feature_id": "clur69pfa00023b6rkftlkucz",
"feature_schema_id": "cltd3vkc201ab07ts1f9dhpkt",
"name": "relationship",
"value": "relationship",
"annotation_kind": "ImageUnidirectionalRelationship",
"classifications": [],
"unidirectional_relationship": {
"source": "clur5tlyq1n2d07dcg9xldg90",
"target": "clur5tlyr1n2l07dc1jsf15qo"
}
}
]
},
"z_order": [
"clur5tlyq1n2d07dcg9xldg90",
"clur5tlyr1n2f07dcf78sa4y5",
"clur5tlyr1n2j07dc50cs4e11",
"clur5tlyr1n2l07dc1jsf15qo",
"clur5tlyr1n2m07dc4x6xhzep",
"clur5tlyr1n2n07dc4qot5gbx",
"clur5tlyr1n2q07dc5joc4k7u",
"clur5tlyr1n2r07dc6ym4d4mc",
"clur5tlyr1n2s07dc739a6kqs",
"clur5tlyr1n2u07dc5wjmdm3v"
]
}
],
"project_details": {
"ontology_id": "cltd3vkbb019q07ts23eedy4o",
"task_id": "a384279a-45c0-4200-b85a-3c18469b3809",
"task_name": "Initial review task",
"batch_id": "3f724e20-da3d-11ee-86a4-0145011952fd",
"batch_name": "image-demo-batch",
"workflow_status": "IN_REVIEW",
"priority": 1,
"consensus_expected_label_count": 1,
"workflow_history": [
{
"action": "Move",
"created_at": "2024-04-08T16:21:26.492+00:00",
"created_by": "aovalle@labelbox.com",
"previous_task_name": "Initial labeling task",
"previous_task_id": "22ee463b-fbe3-0791-8159-94083be9dee3",
"next_task_name": "Initial review task",
"next_task_id": "a384279a-45c0-4200-b85a-3c18469b3809"
},
{
"action": "Move",
"created_at": "2024-04-08T16:21:26.481+00:00",
"created_by": "aovalle@labelbox.com",
"next_task_name": "Initial labeling task",
"next_task_id": "22ee463b-fbe3-0791-8159-94083be9dee3"
}
]
},
"project_tags": []
}
}
}
Sample model run export
{
"data_row": {
"id": "clo78lhhn3dxb07322mnn8plu",
"global_key": "2560px-Kitano_Street_Kobe01s.jpeg",
"row_data": "https://storage.googleapis.com/labelbox-datasets/image_sample_data/2560px-Kitano_Street_Kobe01s5s4110.jpeg",
"details": {
"dataset_id": "clo78lg8f003007321fe52lje",
"dataset_name": "image_prediction_demo",
"created_at": "2023-10-26T13:45:24.929+00:00",
"updated_at": "2023-10-26T13:45:25.965+00:00",
"created_by": "aovalle@labelbox.com"
}
},
"media_attributes": {
"height": 1707,
"width": 2560,
"mime_type": "image/jpeg",
"exif_rotation": "1"
},
"attachments": [],
"metadata_fields": [],
"embeddings": [
{
"id": "cn1cywy1r077807zblvo0m884",
"name": "my_custom_embedding_2048_dimensions",
"dimensions": 2048,
"is_custom": true,
"values": [
{
"value": [
1.1265901290930636,
1.0413733599834287,
1.3041214120015427,
1.893491997791636 ...
]
}
]
},
{
"id": "c200000000000000000000000",
"name": "Image Embedding V2 (CLIP ViT-B/32)",
"dimensions": 512,
"is_custom": false,
"values": [
{
"value": [
-0.4192526042461395,
-0.048827216029167175 ...
]
}
]
}
],
"experiments": {
"a2fb1fec-83fb-0858-2cfb-98f4757eae95": {
"name": "image_model_run_3e5bf56c-31a4-4eed-a731-9ca85e783225",
"runs": {
"a2fb1fec-b8a5-0f96-b61e-0d5643fb8dec": {
"name": "iteration 1",
"run_data_row_id": "42630da9-0dc4-462b-9aa0-5a17e85ff46a",
"labels": [
{
"label_kind": "Default",
"version": "1.0.0",
"id": "clpk3h87e005r14vsp4c33sfq",
"annotations": {
"objects": [
{
"feature_id": "164937d0-7bb2-0f03-8031-34b57799736b",
"feature_schema_id": "clpk3gs0t054y07vig605dopr",
"name": "polygon",
"value": "polygon",
"annotation_kind": "ImagePolygon",
"classifications": [],
"polygon": [
{
"x": 1489.581,
"y": 183.934
},
{
"x": 2278.306,
"y": 256.885
},
{
"x": 2428.197,
"y": 200.437
},
{
"x": 2560.0,
"y": 335.419
},
{
"x": 2557.386,
"y": 503.165
},
{
"x": 2320.596,
"y": 503.103
},
{
"x": 2156.083,
"y": 628.943
},
{
"x": 2161.111,
"y": 785.519
},
{
"x": 2002.115,
"y": 894.647
},
{
"x": 1838.456,
"y": 877.874
},
{
"x": 1436.53,
"y": 874.636
},
{
"x": 1411.403,
"y": 758.579
},
{
"x": 1353.853,
"y": 751.74
},
{
"x": 1345.264,
"y": 453.461
},
{
"x": 1426.011,
"y": 421.129
},
{
"x": 1489.581,
"y": 183.934
}
]
},
{
"feature_id": "2738b5f8-e586-0090-974a-59b6c9b46ee2",
"feature_schema_id": "clpk3gs0t055207vi1baq8bke",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "ImageSegmentationMask",
"classifications": [
{
"feature_id": "9001b75c-bb70-0b40-afbf-9479ecfa9f99",
"feature_schema_id": "clpk3gs0t055307vi90s32rhi",
"name": "sub_free_text",
"value": "sub_free_text",
"text_answer": {
"content": "free text answer"
}
}
],
"mask": {
"url": "https://api.labelbox.com/api/v1/models/a2fb1fec-83fb-0858-2cfb-98f4757eae95/annotations/2738b5f8-e586-0090-974a-59b6c9b46ee2/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6mb7a0dz107xod5w53p61/masks/clpk3h7uk005114vsgru34yw7/index/1",
"color_rgb": [
41,
24,
175
]
}
},
{
"feature_id": "4defcaa5-623f-0589-932a-b703e1116fa4",
"feature_schema_id": "clpk3gs0t055807vi49uy1rcm",
"name": "polyline",
"value": "polyline",
"annotation_kind": "ImagePolyline",
"classifications": [],
"line": [
{
"x": 2534.353,
"y": 249.471
},
{
"x": 2429.492,
"y": 182.092
},
{
"x": 2294.322,
"y": 221.962
},
{
"x": 2224.491,
"y": 180.463
},
{
"x": 2136.123,
"y": 204.716
},
{
"x": 1712.247,
"y": 173.949
},
{
"x": 1703.838,
"y": 84.438
},
{
"x": 1579.772,
"y": 82.61
},
{
"x": 1583.442,
"y": 167.552
},
{
"x": 1478.869,
"y": 164.903
},
{
"x": 1418.941,
"y": 318.149
},
{
"x": 1243.128,
"y": 400.815
},
{
"x": 1022.067,
"y": 319.007
},
{
"x": 892.367,
"y": 379.216
},
{
"x": 670.273,
"y": 364.408
},
{
"x": 613.114,
"y": 288.16
},
{
"x": 377.559,
"y": 238.251
},
{
"x": 368.087,
"y": 185.064
},
{
"x": 246.557,
"y": 167.286
},
{
"x": 236.648,
"y": 285.61
},
{
"x": 90.929,
"y": 326.412
}
]
},
{
"feature_id": "7d129546-45a6-060b-918e-35caec234d31",
"feature_schema_id": "clpk3gs0s054s07vi3ddo8had",
"name": "bbox_with_radio_subclass",
"value": "bbox_with_radio_subclass",
"annotation_kind": "ImageBoundingBox",
"classifications": [
{
"feature_id": "cccba37c-b7a8-095b-8679-dd20aece6f90",
"feature_schema_id": "clpk3gs0s054t07vi17aih47e",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "ea336ea5-041c-02b6-9e8b-6ac1098351b1",
"feature_schema_id": "clpk3gs0s054u07vicrttdlgj",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
],
"bounding_box": {
"top": 933.0,
"left": 541.0,
"height": 191.0,
"width": 330.0
}
},
{
"feature_id": "a3e0496d-3963-0d40-af89-48a907fbb48d",
"feature_schema_id": "clpk3gs0s054q07vi3c30d1be",
"name": "bounding_box",
"value": "bounding_box",
"annotation_kind": "ImageBoundingBox",
"classifications": [],
"bounding_box": {
"top": 977.0,
"left": 1690.0,
"height": 330.0,
"width": 225.0
}
},
{
"feature_id": "f2fc7c1f-7f10-0fc2-b676-fa2591f51a27",
"feature_schema_id": "clpk3gs0t055607vi59sshqn0",
"name": "point",
"value": "point",
"annotation_kind": "ImagePoint",
"classifications": [],
"point": {
"x": 1166.606,
"y": 1441.768
}
},
{
"feature_id": "fb60f23f-8ae1-05b3-a4d8-353839975d59",
"feature_schema_id": "clpk3gs0t055007vi4h7fgm59",
"name": "mask",
"value": "mask",
"annotation_kind": "ImageSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/models/a2fb1fec-83fb-0858-2cfb-98f4757eae95/annotations/fb60f23f-8ae1-05b3-a4d8-353839975d59/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6mb7a0dz107xod5w53p61/masks/clpk3h7uk005114vsgru34yw7/index/1",
"color_rgb": [
186,
26,
40
]
}
}
],
"classifications": [
{
"feature_id": "1be71b85-08da-0ade-bbe4-cfdd431d3e8a",
"feature_schema_id": "clpk3gs0t055g07viaine2doh",
"name": "checklist_question",
"value": "checklist_question",
"checklist_answers": [
{
"feature_id": "0104948e-7be5-032d-80ca-87d052346618",
"feature_schema_id": "clpk3gs0t055j07vi4h3y9wso",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
},
{
"feature_id": "cb587406-5db3-0de2-99f0-cf6e6c344f43",
"feature_schema_id": "clpk3gs0t055h07vi1hp0acnb",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
}
]
},
{
"feature_id": "5b1f4994-1bad-03a1-bf0f-1a0b4682ea25",
"feature_schema_id": "clpk3gs0t055m07vi53ow6auc",
"name": "free_text",
"value": "free_text",
"text_answer": {
"content": "sample text"
}
},
{
"feature_id": "88f8cd4d-e1ca-0add-a7e8-a7349def31bc",
"feature_schema_id": "clpk3gs0t055a07vifk5701ua",
"name": "radio_question",
"value": "radio_question",
"radio_answer": {
"feature_id": "ee141c0d-064f-0de8-b746-e61ac14fbe62",
"feature_schema_id": "clpk3gs0t055d07vibryq8f4l",
"name": "second_radio_answer",
"value": "second_radio_answer",
"classifications": []
}
},
{
"feature_id": "cc8e57d8-5080-0b43-bab1-6e4d78b684de",
"feature_schema_id": "clpk3gs0t055o07vifjxs63xi",
"name": "nested_radio_question",
"value": "nested_radio_question",
"radio_answer": {
"feature_id": "81690da4-5cb5-037f-9a7b-3dd7fefc5481",
"feature_schema_id": "clpk3gs0t055p07vigvkkeii3",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": [
{
"feature_id": "37453082-23dd-0c38-bdcb-90705102bc6e",
"feature_schema_id": "clpk3gs0t055q07vifyau26jb",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "4b014520-7193-04d9-8d69-f800316b60f2",
"feature_schema_id": "clpk3gs0t055r07vi406q70ga",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
]
}
},
{
"feature_id": "f1d18441-41cd-0ff7-9f4f-75384e5a7b51",
"feature_schema_id": "clpk3gs0u055w07vifjngez8o",
"name": "nested_checklist_question",
"value": "nested_checklist_question",
"checklist_answers": [
{
"feature_id": "a3c8745f-8151-0256-8b6d-8ac449797fd7",
"feature_schema_id": "clpk3gs0u055x07vibwdt1pk3",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": [
{
"feature_id": "aa00a3ff-1af4-038a-9be5-97a468656bc5",
"feature_schema_id": "clpk3gs0u055y07vi3srh1g0l",
"name": "sub_checklist_question",
"value": "sub_checklist_question",
"checklist_answers": [
{
"feature_id": "0608a0d7-d9d9-0347-843b-3297e58a25dc",
"feature_schema_id": "clpk3gs0u055z07vifkpvgap4",
"name": "first_sub_checklist_answer",
"value": "first_sub_checklist_answer",
"classifications": []
}
]
}
]
}
]
}
],
"relationships": []
}
}
],
"predictions": [
{
"label_kind": "Default",
"version": "1.0.0",
"id": "clpk3h87e005r14vsp4c33sfq",
"annotations": {
"objects": [
{
"feature_id": "028389ae-93ff-4614-a37e-e867a310371c",
"feature_schema_id": "clpk3gs0s054q07vi3c30d1be",
"name": "bounding_box",
"value": "bounding_box",
"annotation_kind": "ImageBoundingBox",
"classifications": [],
"bounding_box": {
"top": 977.0,
"left": 1690.0,
"height": 330.0,
"width": 225.0
}
},
{
"feature_id": "37415b2f-f376-47b9-8f6a-6429c3efa6af",
"feature_schema_id": "clpk3gs0t054y07vig605dopr",
"name": "polygon",
"value": "polygon",
"annotation_kind": "ImagePolygon",
"classifications": [],
"polygon": [
{
"x": 1489.581,
"y": 183.934
},
{
"x": 2278.306,
"y": 256.885
},
{
"x": 2428.197,
"y": 200.437
},
{
"x": 2560.0,
"y": 335.419
},
{
"x": 2557.386,
"y": 503.165
},
{
"x": 2320.596,
"y": 503.103
},
{
"x": 2156.083,
"y": 628.943
},
{
"x": 2161.111,
"y": 785.519
},
{
"x": 2002.115,
"y": 894.647
},
{
"x": 1838.456,
"y": 877.874
},
{
"x": 1436.53,
"y": 874.636
},
{
"x": 1411.403,
"y": 758.579
},
{
"x": 1353.853,
"y": 751.74
},
{
"x": 1345.264,
"y": 453.461
},
{
"x": 1426.011,
"y": 421.129
},
{
"x": 1489.581,
"y": 183.934
}
]
},
{
"feature_id": "55bee3a4-59c2-4d85-95b3-4584c315037e",
"feature_schema_id": "clpk3gs0t055607vi59sshqn0",
"name": "point",
"value": "point",
"annotation_kind": "ImagePoint",
"classifications": [],
"point": {
"x": 1166.606,
"y": 1441.768
}
},
{
"feature_id": "671736d6-7c30-4399-bec4-a8a1779abf4d",
"feature_schema_id": "clpk3gs0t055007vi4h7fgm59",
"name": "mask",
"value": "mask",
"annotation_kind": "ImageSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/models/a2fb1fec-83fb-0858-2cfb-98f4757eae95/annotations/671736d6-7c30-4399-bec4-a8a1779abf4d/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur6mb7a0dz107xod5w53p61/masks/clpk3gxbq00oi14ok1yykgp85/index/1",
"color_rgb": [
36,
67,
66
]
}
},
{
"feature_id": "7e103ed1-f66f-4cf9-9a36-3e08be3a9648",
"feature_schema_id": "clpk3gs0t055807vi49uy1rcm",
"name": "polyline",
"value": "polyline",
"annotation_kind": "ImagePolyline",
"classifications": [],
"line": [
{
"x": 2534.353,
"y": 249.471
},
{
"x": 2429.492,
"y": 182.092
},
{
"x": 2294.322,
"y": 221.962
},
{
"x": 2224.491,
"y": 180.463
},
{
"x": 2136.123,
"y": 204.716
},
{
"x": 1712.247,
"y": 173.949
},
{
"x": 1703.838,
"y": 84.438
},
{
"x": 1579.772,
"y": 82.61
},
{
"x": 1583.442,
"y": 167.552
},
{
"x": 1478.869,
"y": 164.903
},
{
"x": 1418.941,
"y": 318.149
},
{
"x": 1243.128,
"y": 400.815
},
{
"x": 1022.067,
"y": 319.007
},
{
"x": 892.367,
"y": 379.216
},
{
"x": 670.273,
"y": 364.408
},
{
"x": 613.114,
"y": 288.16
},
{
"x": 377.559,
"y": 238.251
},
{
"x": 368.087,
"y": 185.064
},
{
"x": 246.557,
"y": 167.286
},
{
"x": 236.648,
"y": 285.61
},
{
"x": 90.929,
"y": 326.412
}
]
},
{
"feature_id": "f3a445af-0475-4ad1-892b-fbbcce26073f",
"feature_schema_id": "clpk3gs0s054s07vi3ddo8had",
"name": "bbox_with_radio_subclass",
"value": "bbox_with_radio_subclass",
"annotation_kind": "ImageBoundingBox",
"classifications": [
{
"feature_id": "d8427b71-d516-4686-bc52-660d1133c18d",
"feature_schema_id": "clpk3gs0s054t07vi17aih47e",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "494d3af6-9bf8-477f-8827-dac0dd4c42a3",
"feature_schema_id": "clpk3gs0s054u07vicrttdlgj",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
],
"bounding_box": {
"top": 933.0,
"left": 541.0,
"height": 191.0,
"width": 330.0
}
}
],
"classifications": [
{
"feature_id": "22778fd4-3fd3-48ad-9007-a8f2fc22d664",
"feature_schema_id": "clpk3gs0t055o07vifjxs63xi",
"name": "nested_radio_question",
"value": "nested_radio_question",
"radio_answer": {
"feature_id": "b992d812-7d0b-49b1-82da-14bcff5a76ef",
"feature_schema_id": "clpk3gs0t055p07vigvkkeii3",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": [
{
"feature_id": "877d229e-c877-4aa7-a034-ddde21fa8417",
"feature_schema_id": "clpk3gs0t055q07vifyau26jb",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "803cbe24-7c2f-400c-8d73-fb19bdc5302c",
"feature_schema_id": "clpk3gs0t055r07vi406q70ga",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
]
}
},
{
"feature_id": "2555cb5f-5a90-40f9-987f-7a2899688ad6",
"feature_schema_id": "clpk3gs0t055a07vifk5701ua",
"name": "radio_question",
"value": "radio_question",
"radio_answer": {
"feature_id": "246406e7-162c-43c5-a1ff-ca555be51e55",
"feature_schema_id": "clpk3gs0t055d07vibryq8f4l",
"name": "second_radio_answer",
"value": "second_radio_answer",
"classifications": []
}
},
{
"feature_id": "e4f143b7-5e3d-4f17-8202-efc78cd73f6e",
"feature_schema_id": "clpk3gs0t055m07vi53ow6auc",
"name": "free_text",
"value": "free_text",
"text_answer": {
"content": "sample text"
}
},
{
"feature_id": "ec7d6588-3c15-4fb6-baa1-3508c476bc32",
"feature_schema_id": "clpk3gs0u055w07vifjngez8o",
"name": "nested_checklist_question",
"value": "nested_checklist_question",
"checklist_answers": [
{
"feature_id": "71fd6407-5fc6-43e4-8196-78487f0f9ec8",
"feature_schema_id": "clpk3gs0u055x07vibwdt1pk3",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": [
{
"feature_id": "ab8bf663-3fba-4dd6-9e09-84e0bbd713d0",
"feature_schema_id": "clpk3gs0u055y07vi3srh1g0l",
"name": "sub_checklist_question",
"value": "sub_checklist_question",
"checklist_answers": [
{
"feature_id": "58c98b94-b360-4ceb-b5ea-7765e096c40a",
"feature_schema_id": "clpk3gs0u055z07vifkpvgap4",
"name": "first_sub_checklist_answer",
"value": "first_sub_checklist_answer",
"classifications": []
}
]
}
]
}
]
},
{
"feature_id": "f9107ba1-8020-4b7a-8066-b80167a9cd6a",
"feature_schema_id": "clpk3gs0t055g07viaine2doh",
"name": "checklist_question",
"value": "checklist_question",
"checklist_answers": [
{
"feature_id": "88cc2ed7-8b19-4396-b9ee-36949d23fac7",
"feature_schema_id": "clpk3gs0t055h07vi1hp0acnb",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "b70e6eb1-2ee9-42f3-b47a-a56e764ea4ac",
"feature_schema_id": "clpk3gs0t055j07vi4h3y9wso",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
}
],
"relationships": []
}
}
]
}
}
}
},
"models": {}
}