How to export video annotations and sample export formats.
Export as JSON
# Set the export params to include/exclude certain fields.
export_params= {
"attachments": True,
"metadata_fields": True,
"data_row_details": True,
"project_details": True,
"label_details": True,
"performance_details": True,
"interpolated_frames": True
}
# Note: Filters follow AND logic, so typically using one filter is sufficient.
filters= {
"last_activity_at": ["2000-01-01 00:00:00", "2050-01-01 00:00:00"],
"workflow_status": "<wkf-status>"
}
export_task = project.export(params=export_params, filters=filters)
export_task.wait_till_done()
# Return JSON output strings from export task results/errors, one by one:
# Callback used for JSON Converter
def json_stream_handler(output: lb.BufferedJsonConverterOutput):
print(output.json)
if export_task.has_errors():
export_task.get_buffered_stream(
stream_type=lb.StreamType.ERRORS
).start(stream_handler=lambda error: print(error))
if export_task.has_result():
export_json = export_task.get_buffered_stream(
stream_type=lb.StreamType.RESULT
).start(stream_handler=json_stream_handler)
print("file size: ", export_task.get_total_file_size(stream_type=lb.StreamType.RESULT))
print("line count: ", export_task.get_total_lines(stream_type=lb.StreamType.RESULT))
Annotation export formats
Bounding box
{
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
}
Point
{
"feature_id": "cltd97cag01to14yslakirofl",
"feature_schema_id": "cltd977no037l07x56wfu4536",
"name": "point_video",
"value": "point_video",
"annotation_kind": "VideoPoint",
"classifications": [],
"point": {
"x": 660.134,
"y": 407.926
}
}
Polyline
{
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
Mask
Regardless of which tool is used to create a segmentation mask, the annotation will appear in the following form in the export. Whether you use AutoSegment, the brush tool, the pen tool, or the fill tool, all masks take the same format in exports.
Composite masks
Open this Colab for an interactive tutorial on exporting composite masks.
A composite mask is a cloud-hosted PNG file that contains all raster segmentation mask instances on a labeled data row (one composite mask per label).
This screenshot shows an example composite mask in PNG format. Here, the image has eight segmentation mask instances, each using the schema named "Human".
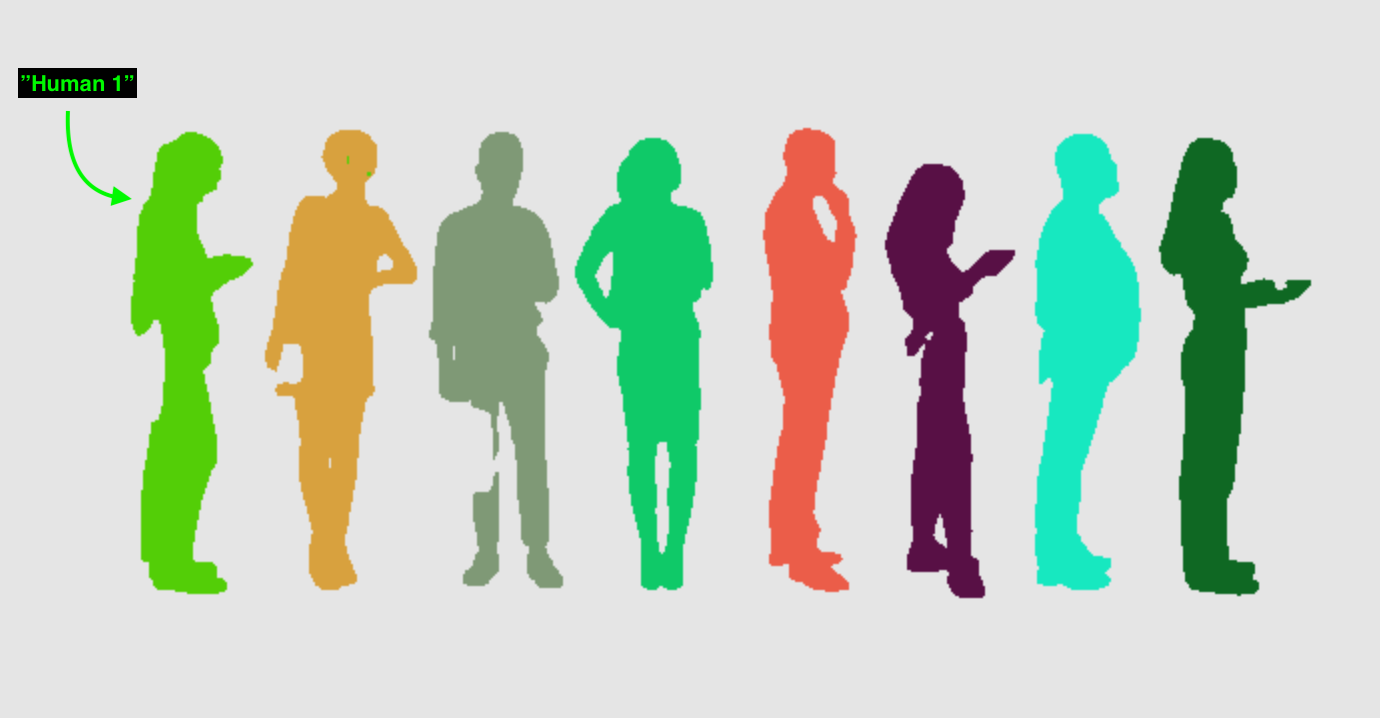
A labeled data row containing 8 instances of a segmentation mask named "Human".
When you export data rows containing segmentation masks, you can use composite_mask.url
to access all segmentation masks on that data row. To access a single instance of a segmentation mask, use the color_rgb
values. The color_rgb
value represents the color of a single annotation or instance mask in a label.
Sample composite masks export
{
"feature_id": "clsivokxg00043b70xuf7x5tz",
"feature_schema_id": "clsgbcaqw000j071t2ga23jnc",
"name": "car",
"value": "car",
"annotation_kind": "ImageSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/clsivnieg050k074i2jqaa0c3/annotations/clsivokxg00043b70xuf7x5tz/index/1/mask"
},
"composite_mask": {
// This URL contains all mask instances on the data row
"url": "https://api.labelbox.com/api/v1/tasks/clsiw88n205mw072ke4n78y5o/masks/clsivok5f00023b70r1a4fgzy",
// This RGB reflects a single mask instance
"color_rgb": [
31,
185,
101
]
}
}
Access mask data
To access the mask data, you must pass your Labelbox API key stored in client.headers
in an API request.
When you grab a URL from the mask annotation in the export, the project_id
and feature_id
will already be in place, as shown above. Here, we provide the framework for structuring a URL with any project ID and feature ID.
import urllib.request
from PIL import Image
# Provide a project ID and feature ID if using instance masks. Alternatively, replace the entire mask_url with a URL grabbed from your export.
project_id = ""
feature_id = ""
mask_url = f"https://api.labelbox.com/api/v1/projects/{project_id}/annotations/{feature_id}/index/1/mask"
# Instead of providing the individual variables, we recommend utilizing the URL from export for composite mask
composite_mask_url = f"https://api.labelbox.com/api/v1/tasks/{task_id}/masks/{composite_mask_id}/index/1"
# Make the API request
req = urllib.request.Request(mask_url, headers=client.headers)
# Optionally, print the image of the mask
image = Image.open(urllib.request.urlopen(req))
image
Classification - Radio
{
"feature_id": "cltd97cag01tf14ysho6tfl7j",
"feature_schema_id": "cltd977np038907x5ah8l9ha7",
"name": "radio_class",
"value": "radio_class",
"radio_answer": {
"feature_id": "cltd97cag01tg14ys171a3qqr",
"feature_schema_id": "cltd977np038a07x515axagkl",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": []
}
}
Classification - Checklist
{
"feature_id": "cltd97cag01tq14ysb4m3nyjo",
"feature_schema_id": "cltd977nq039107x5dsml2knm",
"name": "checklist_class_global",
"value": "checklist_class_global",
"checklist_answers": [
{
"feature_id": "cltd97cag01tr14ysozjbx0c6",
"feature_schema_id": "cltd977nq039207x5ei1605s1",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "cltd97cag01ts14ys4vvxihnw",
"feature_schema_id": "cltd977nq039407x50qs3g61l",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
}
Classification - Free-form text
{
"feature_id": "cltd97cag01u314ysx1h72f0h",
"feature_schema_id": "cltd977nq039707x535ufeo39",
"name": "free_text",
"value": "free_text",
"text_answer": {
"content": "sample text"
}
}
Sample project export
{
"data_row": {
"id": "clp1jolki07uy0725flku4bmv",
"global_key": "sample-video-jellyfish.mp4",
"row_data": "https://storage.googleapis.com/labelbox-datasets/video-sample-data/sample-video-2.mp4",
"details": {
"dataset_id": "clp1jokqp000p07307gtfy1w3",
"dataset_name": "video_demo_dataset",
"created_at": "2023-11-16T18:48:51.208+00:00",
"updated_at": "2023-11-16T18:48:51.801+00:00",
"last_activity_at": "2024-04-08T17:09:54.000+00:00",
"created_by": "[email protected]"
}
},
"media_attributes": {
"height": 1080,
"width": 1920,
"mime_type": "video/mp4",
"frame_rate": 10,
"frame_count": 100
},
"attachments": [],
"metadata_fields": [],
"embeddings": [
{
"id": "c400000000000000000000000",
"name": "Video Embedding V2 (Google MultiModal)",
"dimensions": 1408,
"is_custom": false,
"values": [
{
"value": [
-0.00489852577,
0.0287129097 ...
]
}
]
}
],
"projects": {
"cltd977uu016y08xyggep8aiv": {
"name": "Video Annotation Import Demo",
"labels": [
{
"label_kind": "Video",
"version": "1.0.0",
"id": "cltd97caz01ub14ysq8rf4x8w",
"label_details": {
"created_at": "2024-03-04T18:07:33.000+00:00",
"updated_at": "2024-03-04T18:07:33.000+00:00",
"created_by": "[email protected]",
"content_last_updated_at": "2024-03-04T18:07:33.369+00:00",
"reviews": []
},
"performance_details": {
"seconds_to_create": 0,
"seconds_to_review": 0,
"skipped": false
},
"annotations": {
"frames": {
"29": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": [
{
"feature_id": "cltd97cag01tc14yspm0lev2d",
"feature_schema_id": "cltd977np038307x55dlf3rgs",
"name": "checklist_class",
"value": "checklist_class",
"checklist_answers": [
{
"feature_id": "cltd97cag01td14ysxw2n8xkz",
"feature_schema_id": "cltd977np038407x51hcvdad7",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "cltd97cag01te14ys1jdf1fku",
"feature_schema_id": "cltd977np038607x58epu4nb5",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
}
]
},
"35": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": [
{
"feature_id": "cltd97cag01tc14yspm0lev2d",
"feature_schema_id": "cltd977np038307x55dlf3rgs",
"name": "checklist_class",
"value": "checklist_class",
"checklist_answers": [
{
"feature_id": "cltd97cag01td14ysxw2n8xkz",
"feature_schema_id": "cltd977np038407x51hcvdad7",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "cltd97cag01te14ys1jdf1fku",
"feature_schema_id": "cltd977np038607x58epu4nb5",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
}
]
},
"9": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.00000000000001
},
{
"x": 100.00000000000001,
"y": 190.0
}
]
}
},
"classifications": [
{
"feature_id": "cltd97cag01tf14ysho6tfl7j",
"feature_schema_id": "cltd977np038907x5ah8l9ha7",
"name": "radio_class",
"value": "radio_class",
"radio_answer": {
"feature_id": "cltd97cag01tg14ys171a3qqr",
"feature_schema_id": "cltd977np038a07x515axagkl",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": []
}
}
]
},
"15": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": [
{
"feature_id": "cltd97cag01tf14ysho6tfl7j",
"feature_schema_id": "cltd977np038907x5ah8l9ha7",
"name": "radio_class",
"value": "radio_class",
"radio_answer": {
"feature_id": "cltd97cag01tg14ys171a3qqr",
"feature_schema_id": "cltd977np038a07x515axagkl",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": []
}
}
]
},
"13": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01ti14ys8wedf89d": {
"feature_id": "cltd97cag01ti14ys8wedf89d",
"feature_schema_id": "cltd977np037r07x512iteflz",
"name": "bbox_class",
"value": "bbox_class",
"annotation_kind": "VideoBoundingBox",
"classifications": [
{
"feature_id": "cltd97cag01tj14ysz455nenr",
"feature_schema_id": "cltd977np037s07x5csbodf5f",
"name": "checklist_class",
"value": "checklist_class",
"radio_answer": {
"feature_id": "cltd97cag01tl14ysnbe8mw75",
"feature_schema_id": "cltd977np037v07x54esmdmws",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
}
],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"14": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0000000000001,
"left": 1371.0,
"height": 418.9999999999999,
"width": 505.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"16": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"17": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01to14yslakirofl": {
"feature_id": "cltd97cag01to14yslakirofl",
"feature_schema_id": "cltd977no037l07x56wfu4536",
"name": "point_video",
"value": "point_video",
"annotation_kind": "VideoPoint",
"classifications": [],
"point": {
"x": 660.134,
"y": 407.926
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"18": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"19": {
"objects": {
"cltd97cag01th14ys6fyyxyk9": {
"feature_id": "cltd97cag01th14ys6fyyxyk9",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"10": {
"objects": {
"cltd97cag01ti14ys8wedf89d": {
"feature_id": "cltd97cag01ti14ys8wedf89d",
"feature_schema_id": "cltd977np037r07x512iteflz",
"name": "bbox_class",
"value": "bbox_class",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"11": {
"objects": {
"cltd97cag01ti14ys8wedf89d": {
"feature_id": "cltd97cag01ti14ys8wedf89d",
"feature_schema_id": "cltd977np037r07x512iteflz",
"name": "bbox_class",
"value": "bbox_class",
"annotation_kind": "VideoBoundingBox",
"classifications": [
{
"feature_id": "cltd97cag01tj14ysz455nenr",
"feature_schema_id": "cltd977np037s07x5csbodf5f",
"name": "checklist_class",
"value": "checklist_class",
"radio_answer": {
"feature_id": "cltd97cag01tk14ysejipk2y6",
"feature_schema_id": "cltd977np037t07x5966ebxpe",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
}
}
],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"12": {
"objects": {
"cltd97cag01ti14ys8wedf89d": {
"feature_id": "cltd97cag01ti14ys8wedf89d",
"feature_schema_id": "cltd977np037r07x512iteflz",
"name": "bbox_class",
"value": "bbox_class",
"annotation_kind": "VideoBoundingBox",
"classifications": [
{
"feature_id": "cltd97cag01tj14ysz455nenr",
"feature_schema_id": "cltd977np037s07x5csbodf5f",
"name": "checklist_class",
"value": "checklist_class",
"radio_answer": {
"feature_id": "cltd97cag01tk14ysejipk2y6",
"feature_schema_id": "cltd977np037t07x5966ebxpe",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
}
}
],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"22": {
"objects": {
"cltd97cag01tm14ys8tm4x7yl": {
"feature_id": "cltd97cag01tm14ys8tm4x7yl",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tn14yspqge5yph": {
"feature_id": "cltd97cag01tn14yspqge5yph",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
}
},
"classifications": []
},
"23": {
"objects": {
"cltd97cag01tm14ys8tm4x7yl": {
"feature_id": "cltd97cag01tm14ys8tm4x7yl",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0000000000002
}
},
"cltd97cag01tn14yspqge5yph": {
"feature_id": "cltd97cag01tn14yspqge5yph",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.00000000000006
}
}
},
"classifications": []
},
"24": {
"objects": {
"cltd97cag01tm14ys8tm4x7yl": {
"feature_id": "cltd97cag01tm14ys8tm4x7yl",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tn14yspqge5yph": {
"feature_id": "cltd97cag01tn14yspqge5yph",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
},
"cltd97cag01u514ys2bfg9vvo": {
"feature_id": "cltd97cag01u514ys2bfg9vvo",
"feature_schema_id": "cltd977np037p07x55hu71x6k",
"name": "video_mask",
"value": "video_mask",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cag01u514ys2bfg9vvo/index/24/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/24",
"color_rgb": [
76,
104,
177
]
}
},
"cltd97cah01u614ysvntxcyub": {
"feature_id": "cltd97cah01u614ysvntxcyub",
"feature_schema_id": "cltd977np037z07x5gclv41y4",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01u614ysvntxcyub/index/24/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/24",
"color_rgb": [
233,
218,
94
]
}
},
"cltd97cah01u714ysbqbvqs6b": {
"feature_id": "cltd97cah01u714ysbqbvqs6b",
"feature_schema_id": "cltd977np037p07x55hu71x6k",
"name": "video_mask",
"value": "video_mask",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01u714ysbqbvqs6b/index/24/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/24",
"color_rgb": [
83,
152,
103
]
}
}
},
"classifications": []
},
"25": {
"objects": {
"cltd97cag01tm14ys8tm4x7yl": {
"feature_id": "cltd97cag01tm14ys8tm4x7yl",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tn14yspqge5yph": {
"feature_id": "cltd97cag01tn14yspqge5yph",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 99.99999999999999
},
{
"x": 99.99999999999999,
"y": 189.99999999999997
}
]
}
},
"classifications": []
},
"26": {
"objects": {
"cltd97cag01tm14ys8tm4x7yl": {
"feature_id": "cltd97cag01tm14ys8tm4x7yl",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tn14yspqge5yph": {
"feature_id": "cltd97cag01tn14yspqge5yph",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
},
"cltd97cah01ua14ysr6u1sh7w": {
"feature_id": "cltd97cah01ua14ysr6u1sh7w",
"feature_schema_id": "cltd977np037z07x5gclv41y4",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01ua14ysr6u1sh7w/index/26/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/26",
"color_rgb": [
226,
211,
41
]
}
}
},
"classifications": []
},
"27": {
"objects": {
"cltd97cag01tm14ys8tm4x7yl": {
"feature_id": "cltd97cag01tm14ys8tm4x7yl",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 617.0,
"left": 1371.0,
"height": 419.0,
"width": 505.0
}
},
"cltd97cag01tn14yspqge5yph": {
"feature_id": "cltd97cag01tn14yspqge5yph",
"feature_schema_id": "cltd977no037j07x5d82dacbc",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
},
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"5": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"6": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"7": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.00000000000003
}
]
}
},
"classifications": []
},
"8": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"20": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
},
"cltd97cag01u514ys2bfg9vvo": {
"feature_id": "cltd97cag01u514ys2bfg9vvo",
"feature_schema_id": "cltd977np037p07x55hu71x6k",
"name": "video_mask",
"value": "video_mask",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cag01u514ys2bfg9vvo/index/20/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/20",
"color_rgb": [
76,
104,
177
]
}
},
"cltd97cah01u614ysvntxcyub": {
"feature_id": "cltd97cah01u614ysvntxcyub",
"feature_schema_id": "cltd977np037z07x5gclv41y4",
"name": "mask_with_text_subclass",
"value": "mask_with_text_subclass",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01u614ysvntxcyub/index/20/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/20",
"color_rgb": [
233,
218,
94
]
}
},
"cltd97cah01u714ysbqbvqs6b": {
"feature_id": "cltd97cah01u714ysbqbvqs6b",
"feature_schema_id": "cltd977np037p07x55hu71x6k",
"name": "video_mask",
"value": "video_mask",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01u714ysbqbvqs6b/index/20/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/20",
"color_rgb": [
83,
152,
103
]
}
}
},
"classifications": []
},
"28": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"30": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"31": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"32": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"33": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"34": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"36": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"37": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"38": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"39": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"40": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"41": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"42": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"43": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"44": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"45": {
"objects": {
"cltd97cag01tp14ysz3my848t": {
"feature_id": "cltd97cag01tp14ysz3my848t",
"feature_schema_id": "cltd977np037n07x5d1buckz9",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
}
]
}
},
"classifications": []
},
"1": {
"objects": {
"cltd97cah01u814ys0k41cttu": {
"feature_id": "cltd97cah01u814ys0k41cttu",
"feature_schema_id": "cltd977np037p07x55hu71x6k",
"name": "video_mask",
"value": "video_mask",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01u814ys0k41cttu/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/1",
"color_rgb": [
169,
248,
152
]
}
},
"cltd97cah01u914ysy7t58lvy": {
"feature_id": "cltd97cah01u914ysy7t58lvy",
"feature_schema_id": "cltd977np037p07x55hu71x6k",
"name": "video_mask",
"value": "video_mask",
"annotation_kind": "VideoSegmentationMask",
"classifications": [],
"mask": {
"url": "https://api.labelbox.com/api/v1/projects/cltd977uu016y08xyggep8aiv/annotations/cltd97cah01u914ysy7t58lvy/index/1/mask"
},
"composite_mask": {
"url": "https://api.labelbox.com/api/v1/tasks/clur7t5d501i707wfawrp050b/masks/cltd97a7h01tb14ysldafd9es/index/1",
"color_rgb": [
227,
135,
126
]
}
}
},
"classifications": []
}
},
"segments": {
"cltd97cag01td14ysxw2n8xkz": [
[
29,
35
]
],
"cltd97cag01te14ys1jdf1fku": [
[
29,
35
]
],
"cltd97cag01tg14ys171a3qqr": [
[
9,
15
]
],
"cltd97cag01th14ys6fyyxyk9": [
[
13,
19
]
],
"cltd97cag01ti14ys8wedf89d": [
[
10,
13
]
],
"cltd97cag01tm14ys8tm4x7yl": [
[
22,
27
]
],
"cltd97cag01tn14yspqge5yph": [
[
22,
27
]
],
"cltd97cag01to14yslakirofl": [
[
17,
17
]
],
"cltd97cag01tp14ysz3my848t": [
[
5,
20
],
[
24,
45
]
]
},
"key_frame_feature_map": {
"cltd97cag01tc14yspm0lev2d": [
29,
35
],
"cltd97cag01tp14ysz3my848t": [
24,
5,
20,
45
],
"cltd97cag01tf14ysho6tfl7j": [
9,
15
],
"cltd97cag01th14ys6fyyxyk9": [
13,
19
],
"cltd97cag01ti14ys8wedf89d": [
13,
10
],
"cltd97cag01to14yslakirofl": [
17
],
"cltd97cag01tm14ys8tm4x7yl": [
22,
27
],
"cltd97cag01tn14yspqge5yph": [
22,
27
],
"cltd97cag01u514ys2bfg9vvo": [
24,
20
],
"cltd97cah01u614ysvntxcyub": [
24,
20
],
"cltd97cah01u714ysbqbvqs6b": [
24,
20
],
"cltd97cah01ua14ysr6u1sh7w": [
26
],
"cltd97cah01u814ys0k41cttu": [
1
],
"cltd97cah01u914ysy7t58lvy": [
1
]
},
"classifications": [
{
"feature_id": "cltd97cag01tq14ysb4m3nyjo",
"feature_schema_id": "cltd977nq039107x5dsml2knm",
"name": "checklist_class_global",
"value": "checklist_class_global",
"checklist_answers": [
{
"feature_id": "cltd97cag01tr14ysozjbx0c6",
"feature_schema_id": "cltd977nq039207x5ei1605s1",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
},
{
"feature_id": "cltd97cag01ts14ys4vvxihnw",
"feature_schema_id": "cltd977nq039407x50qs3g61l",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
}
]
},
{
"feature_id": "cltd97cag01tt14yscmqh2izy",
"feature_schema_id": "cltd977nq038v07x57qz18lnj",
"name": "radio_class_global",
"value": "radio_class_global",
"radio_answer": {
"feature_id": "cltd97cag01tu14ysq046ad6p",
"feature_schema_id": "cltd977nq038w07x5bxw3exp9",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": []
}
},
{
"feature_id": "cltd97cag01tv14ys1y3u4jre",
"feature_schema_id": "cltd977nq038n07x52t8s0j1f",
"name": "nested_checklist_question",
"value": "nested_checklist_question",
"checklist_answers": [
{
"feature_id": "cltd97cag01tw14ysieenbrzy",
"feature_schema_id": "cltd977nq038o07x528j39vc4",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": [
{
"feature_id": "cltd97cag01tx14ysaqs15mjh",
"feature_schema_id": "cltd977nq038p07x5bfuf7v56",
"name": "sub_checklist_question",
"value": "sub_checklist_question",
"checklist_answers": [
{
"feature_id": "cltd97cag01ty14yshujgka3h",
"feature_schema_id": "cltd977nq038q07x52m1h32mj",
"name": "first_sub_checklist_answer",
"value": "first_sub_checklist_answer",
"classifications": []
}
]
}
]
}
]
},
{
"feature_id": "cltd97cag01tz14ys73p2w3oa",
"feature_schema_id": "cltd977nq038f07x5bgrc2fm6",
"name": "nested_radio_question",
"value": "nested_radio_question",
"radio_answer": {
"feature_id": "cltd97cag01u014ysho9dljue",
"feature_schema_id": "cltd977nq038g07x50ewqbbi3",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": [
{
"feature_id": "cltd97cag01u114ysktcruoro",
"feature_schema_id": "cltd977nq038h07x59zx07wfe",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "cltd97cag01u214ysxi71u4qi",
"feature_schema_id": "cltd977nq038i07x5et0717w6",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
]
}
},
{
"feature_id": "cltd97cag01u314ysx1h72f0h",
"feature_schema_id": "cltd977nq039707x535ufeo39",
"name": "free_text",
"value": "free_text",
"text_answer": {
"content": "sample text"
}
}
]
}
}
],
"project_details": {
"ontology_id": "cltd977mx037i07x52amua8iw",
"task_name": "Done",
"batch_id": "1026dd60-da52-11ee-9774-cfce344359c8",
"batch_name": "first-batch-video-demo2",
"workflow_status": "DONE",
"priority": 5,
"consensus_expected_label_count": 1,
"workflow_history": []
}
}
}
}
Sample model run export
{
"data_row": {
"id": "cldtd2nai107407y5979xbu97",
"global_key": "3548cfa1-1a42-40f9-a246-2aa7c696dcbe",
"row_data": "https://storage.googleapis.com/labelbox-datasets/video-sample-data/sample-video-2.mp4",
"details": {
"dataset_id": "cldtd2mox11dz07wh3gkk9awx",
"dataset_name": "video_prediction_demo",
"created_at": "2023-02-06T22:04:54.000+00:00",
"updated_at": "2023-02-06T22:04:54.000+00:00",
"created_by": "[email protected]"
}
},
"media_attributes": {
"height": 1080,
"width": 1920,
"mime_type": "video/mp4",
"frame_rate": 10,
"frame_count": 100
},
"attachments": [],
"metadata_fields": [],
"experiments": {
"a088408e-81af-0152-f2ca-0b870c7a2bfa": {
"name": "video_model_run_8d7dbe3d-30e0-4c9c-becd-26afb2386bc9",
"runs": {
"a088408e-ce36-09c1-cbd9-d43c677f7773": {
"name": "iteration 1",
"run_data_row_id": "999a247e-0b57-415e-af7e-d3a87cc6559b",
"labels": [
{
"label_kind": "Video",
"version": "1.0.0",
"id": "cldteelzh08us1331q4pzj2ke",
"annotations": {
"frames": {
"17": {
"objects": {
"12a89fb9-73e4-4c5e-93c1-a8727e51c122": {
"feature_id": "12a89fb9-73e4-4c5e-93c1-a8727e51c122",
"feature_schema_id": "cldtd2nmm107i07y53ii0d6ly",
"name": "point_video",
"value": "point_video",
"annotation_kind": "VideoPoint",
"classifications": [],
"point": {
"x": 660.134,
"y": 407.926
}
}
},
"classifications": []
},
"5": {
"objects": {
"878b4842-d57b-43e4-8327-a0c5295f2a84": {
"feature_id": "878b4842-d57b-43e4-8327-a0c5295f2a84",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
},
{
"x": 190.0,
"y": 220.0
}
]
},
"9cd6cd8e-0511-42a9-bc2f-726cf5c8f983": {
"feature_id": "9cd6cd8e-0511-42a9-bc2f-726cf5c8f983",
"feature_schema_id": "cldtd2nml107a07y51qtydeoj",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
}
},
"classifications": []
},
"12": {
"objects": {
"878b4842-d57b-43e4-8327-a0c5295f2a84": {
"feature_id": "878b4842-d57b-43e4-8327-a0c5295f2a84",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 280.0
},
{
"x": 300.0,
"y": 380.0
},
{
"x": 400.0,
"y": 460.0
}
]
}
},
"classifications": []
},
"20": {
"objects": {
"878b4842-d57b-43e4-8327-a0c5295f2a84": {
"feature_id": "878b4842-d57b-43e4-8327-a0c5295f2a84",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 180.0
},
{
"x": 100.0,
"y": 200.0
},
{
"x": 200.0,
"y": 260.0
}
]
}
},
"classifications": []
},
"24": {
"objects": {
"878b4842-d57b-43e4-8327-a0c5295f2a84": {
"feature_id": "878b4842-d57b-43e4-8327-a0c5295f2a84",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 300.0,
"y": 310.0
},
{
"x": 330.0,
"y": 430.0
}
]
}
},
"classifications": []
},
"45": {
"objects": {
"878b4842-d57b-43e4-8327-a0c5295f2a84": {
"feature_id": "878b4842-d57b-43e4-8327-a0c5295f2a84",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 600.0,
"y": 810.0
},
{
"x": 900.0,
"y": 930.0
}
]
}
},
"classifications": []
},
"1": {
"objects": {
"9cd6cd8e-0511-42a9-bc2f-726cf5c8f983": {
"feature_id": "9cd6cd8e-0511-42a9-bc2f-726cf5c8f983",
"feature_schema_id": "cldtd2nml107a07y51qtydeoj",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
}
},
"classifications": []
}
},
"segments": {
"12a89fb9-73e4-4c5e-93c1-a8727e51c122": [
[
17,
17
]
],
"878b4842-d57b-43e4-8327-a0c5295f2a84": [
[
5,
5
],
[
12,
12
],
[
20,
20
],
[
24,
24
],
[
45,
45
]
],
"9cd6cd8e-0511-42a9-bc2f-726cf5c8f983": [
[
5,
5
],
[
1,
1
]
]
},
"key_frame_feature_map": {
"12a89fb9-73e4-4c5e-93c1-a8727e51c122": [
17
],
"878b4842-d57b-43e4-8327-a0c5295f2a84": [
5,
12,
20,
24,
45
],
"9cd6cd8e-0511-42a9-bc2f-726cf5c8f983": [
5,
1
]
},
"classifications": [
{
"feature_id": "3644a35a-8aaa-4905-a2d2-9abac1f3dacb",
"feature_schema_id": "cldtd2nmm107s07y57ddy5wnn",
"name": "checklist_question",
"value": "checklist_question",
"checklist_answers": [
{
"feature_id": "86805980-7632-4dd0-9669-299730927326",
"feature_schema_id": "cldtd2nmm107v07y5cmixhywh",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
},
{
"feature_id": "c5bfb5c1-aa9a-4a6a-b671-be7c20df4b19",
"feature_schema_id": "cldtd2nmm107t07y5cqdw94z7",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
}
]
},
{
"feature_id": "3d302a82-cc91-41d9-8c69-0f1e2e66ea71",
"feature_schema_id": "cldtd2nmm107m07y5h45sg6av",
"name": "radio_question",
"value": "radio_question",
"radio_answer": {
"feature_id": "dab3bcfa-6c44-4f28-945b-1bc324edfab1",
"feature_schema_id": "cldtd2nmm107p07y542ff3hvd",
"name": "second_radio_answer",
"value": "second_radio_answer",
"classifications": []
}
},
{
"feature_id": "6dede674-18a6-4b95-abdd-13b3713f994a",
"feature_schema_id": "cldtd2nmm108607y5ghou4ma6",
"name": "nested_checklist_question",
"value": "nested_checklist_question",
"checklist_answers": [
{
"feature_id": "9f748713-ffb5-44db-a16e-396676dab6bc",
"feature_schema_id": "cldtd2nmm108707y50shn5996",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": [
{
"feature_id": "ca1f2173-38c6-4c23-96ba-8a3feb54e2c2",
"feature_schema_id": "cldtd2nmm108807y52ps9d5m4",
"name": "sub_checklist_question",
"value": "sub_checklist_question",
"checklist_answers": [
{
"feature_id": "2181d943-8a6a-4aef-aae0-4c28146e9008",
"feature_schema_id": "cldtd2nmm108907y5269ua7bz",
"name": "first_sub_checklist_answer",
"value": "first_sub_checklist_answer",
"classifications": []
}
]
}
]
}
]
},
{
"feature_id": "b72dd664-93fc-46d1-8bd8-8dfe1fd2d7ef",
"feature_schema_id": "cldtd2nmm107y07y5fvhkb6c7",
"name": "nested_radio_question",
"value": "nested_radio_question",
"radio_answer": {
"feature_id": "5fc17086-601e-42e5-a5b1-a28eb96841ab",
"feature_schema_id": "cldtd2nmm107z07y59j8n62ym",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": [
{
"feature_id": "a74e9958-345f-4b42-925d-9d5938011cf6",
"feature_schema_id": "cldtd2nmm108007y5gs1y5eqv",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "734a2cf1-571d-4855-869e-d92ff20a4cb4",
"feature_schema_id": "cldtd2nmm108107y53dvreuv2",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
]
}
}
]
}
}
],
"predictions": [
{
"label_kind": "Video",
"version": "1.0.0",
"id": "cldteelzh08us1331q4pzj2ke",
"annotations": {
"frames": {
"5": {
"objects": {
"1306f719-d588-4154-8335-792347bfdee2": {
"feature_id": "1306f719-d588-4154-8335-792347bfdee2",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 100.0
},
{
"x": 100.0,
"y": 190.0
},
{
"x": 190.0,
"y": 220.0
}
]
},
"a505f426-5a2e-4dea-b4fe-ab913e1d2a99": {
"feature_id": "a505f426-5a2e-4dea-b4fe-ab913e1d2a99",
"feature_schema_id": "cldtd2nml107a07y51qtydeoj",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
}
},
"classifications": []
},
"12": {
"objects": {
"1306f719-d588-4154-8335-792347bfdee2": {
"feature_id": "1306f719-d588-4154-8335-792347bfdee2",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 280.0
},
{
"x": 300.0,
"y": 380.0
},
{
"x": 400.0,
"y": 460.0
}
]
}
},
"classifications": []
},
"20": {
"objects": {
"1306f719-d588-4154-8335-792347bfdee2": {
"feature_id": "1306f719-d588-4154-8335-792347bfdee2",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 680.0,
"y": 180.0
},
{
"x": 100.0,
"y": 200.0
},
{
"x": 200.0,
"y": 260.0
}
]
}
},
"classifications": []
},
"24": {
"objects": {
"1306f719-d588-4154-8335-792347bfdee2": {
"feature_id": "1306f719-d588-4154-8335-792347bfdee2",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 300.0,
"y": 310.0
},
{
"x": 330.0,
"y": 430.0
}
]
}
},
"classifications": []
},
"45": {
"objects": {
"1306f719-d588-4154-8335-792347bfdee2": {
"feature_id": "1306f719-d588-4154-8335-792347bfdee2",
"feature_schema_id": "cldtd2nmm107k07y577q807az",
"name": "line_video_frame",
"value": "line_video_frame",
"annotation_kind": "VideoPolyline",
"classifications": [],
"line": [
{
"x": 600.0,
"y": 810.0
},
{
"x": 900.0,
"y": 930.0
}
]
}
},
"classifications": []
},
"17": {
"objects": {
"89b4636f-08cc-4fa2-832b-18bcda636722": {
"feature_id": "89b4636f-08cc-4fa2-832b-18bcda636722",
"feature_schema_id": "cldtd2nmm107i07y53ii0d6ly",
"name": "point_video",
"value": "point_video",
"annotation_kind": "VideoPoint",
"classifications": [],
"point": {
"x": 660.134,
"y": 407.926
}
}
},
"classifications": []
},
"1": {
"objects": {
"a505f426-5a2e-4dea-b4fe-ab913e1d2a99": {
"feature_id": "a505f426-5a2e-4dea-b4fe-ab913e1d2a99",
"feature_schema_id": "cldtd2nml107a07y51qtydeoj",
"name": "bbox_video",
"value": "bbox_video",
"annotation_kind": "VideoBoundingBox",
"classifications": [],
"bounding_box": {
"top": 146.0,
"left": 98.0,
"height": 382.0,
"width": 341.0
}
}
},
"classifications": []
}
},
"segments": {
"1306f719-d588-4154-8335-792347bfdee2": [
[
5,
5
],
[
12,
12
],
[
20,
20
],
[
24,
24
],
[
45,
45
]
],
"a505f426-5a2e-4dea-b4fe-ab913e1d2a99": [
[
5,
5
],
[
1,
1
]
],
"89b4636f-08cc-4fa2-832b-18bcda636722": [
[
17,
17
]
]
},
"key_frame_feature_map": {
"1306f719-d588-4154-8335-792347bfdee2": [
5,
12,
20,
24,
45
],
"a505f426-5a2e-4dea-b4fe-ab913e1d2a99": [
5,
1
],
"89b4636f-08cc-4fa2-832b-18bcda636722": [
17
]
},
"classifications": [
{
"feature_id": "5deccc93-922a-4fcd-bf70-0bd90249ed65",
"feature_schema_id": "cldtd2nmm108607y5ghou4ma6",
"name": "nested_checklist_question",
"value": "nested_checklist_question",
"checklist_answers": [
{
"feature_id": "3d9f3952-c303-42ed-91ba-b112927029c9",
"feature_schema_id": "cldtd2nmm108707y50shn5996",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": [
{
"feature_id": "fe4d0f5f-99e6-4abd-9401-b0ad04fe13ed",
"feature_schema_id": "cldtd2nmm108807y52ps9d5m4",
"name": "sub_checklist_question",
"value": "sub_checklist_question",
"checklist_answers": [
{
"feature_id": "05fca900-787a-4774-a011-0b6931d9a012",
"feature_schema_id": "cldtd2nmm108907y5269ua7bz",
"name": "first_sub_checklist_answer",
"value": "first_sub_checklist_answer",
"classifications": []
}
]
}
]
}
]
},
{
"feature_id": "7a92c014-48e1-4367-8ff9-4ec146d9968e",
"feature_schema_id": "cldtd2nmm107y07y5fvhkb6c7",
"name": "nested_radio_question",
"value": "nested_radio_question",
"radio_answer": {
"feature_id": "9f35c500-be1d-4693-ae9a-71507931d558",
"feature_schema_id": "cldtd2nmm107z07y59j8n62ym",
"name": "first_radio_answer",
"value": "first_radio_answer",
"classifications": [
{
"feature_id": "d1269040-bf2c-4e8c-8fa6-58c3d6a7e343",
"feature_schema_id": "cldtd2nmm108007y5gs1y5eqv",
"name": "sub_radio_question",
"value": "sub_radio_question",
"radio_answer": {
"feature_id": "2fecd9bc-98ca-49bd-8db4-cb9d1be6a63d",
"feature_schema_id": "cldtd2nmm108107y53dvreuv2",
"name": "first_sub_radio_answer",
"value": "first_sub_radio_answer",
"classifications": []
}
}
]
}
},
{
"feature_id": "95085369-6add-4ce1-9641-d6b690490eb3",
"feature_schema_id": "cldtd2nmm107s07y57ddy5wnn",
"name": "checklist_question",
"value": "checklist_question",
"checklist_answers": [
{
"feature_id": "53a98fa1-19ea-4541-b42f-8df7f7e3b2fc",
"feature_schema_id": "cldtd2nmm107v07y5cmixhywh",
"name": "second_checklist_answer",
"value": "second_checklist_answer",
"classifications": []
},
{
"feature_id": "6383f391-7ca6-4d99-a855-cdc2e2afa811",
"feature_schema_id": "cldtd2nmm107t07y5cqdw94z7",
"name": "first_checklist_answer",
"value": "first_checklist_answer",
"classifications": []
}
]
},
{
"feature_id": "af2f95fe-6ce6-4e3a-83a8-fd11b1ae0cdc",
"feature_schema_id": "cldtd2nmm107m07y5h45sg6av",
"name": "radio_question",
"value": "radio_question",
"radio_answer": {
"feature_id": "71d910d5-df6b-4def-b68e-caced48dd25c",
"feature_schema_id": "cldtd2nmm107p07y542ff3hvd",
"name": "second_radio_answer",
"value": "second_radio_answer",
"classifications": []
}
}
]
}
}
]
}
}
}
},
"models": {},
"embeddings": [
{
"id": "c400000000000000000000000",
"name": "Video Embedding V2 (Google MultiModal)",
"dimensions": 1408,
"is_custom": false,
"values": [
{
"value": [
-0.00489852577,
0.0287129097 ...
]
}
]
}
]
}